The aviation industry is constantly striving to improve efficiency and reduce operational costs, particularly in the Maintenance, Repair, and Overhaul (MRO) sector. At ePlaneAI, we offer advanced solutions utilizing What-if Analysis powered by Decision Tree models to understand potential scenarios in MRO operations, allowing airlines and MRO providers to make informed decisions that reduce aircraft downtime and optimize resources. Our solutions include building custom, data-intensive machine learning models and dashboards or integrating with existing cloud-based dashboard solutions. In this article, we will explore how Decision Trees can be utilized for What-if Analysis, focusing on practical applications in aviation MRO, complete with detailed code examples and increased complexity to reflect real-world scenarios.
The Importance of What-if Analysis in MRO Decision-Making
In the aviation industry, maintenance schedules, repair strategies, and resource allocation must be handled with extreme care. What-if Analysis allows MRO teams to anticipate the outcomes of different maintenance decisions, such as changing inspection intervals or shifting repair priorities. When paired with Decision Tree models, What-if Analysis offers:
- Scenario Exploration: Evaluate different maintenance strategies to determine which actions minimize aircraft downtime.
- Resource Optimization: Identify how changes in technician assignments, spare part availability, and equipment affect operational efficiency.
- Risk Mitigation: Visualize the outcomes of different risk mitigation strategies, enabling data-driven decision-making to minimize costs and improve safety.
Granular Scenarios: Optimizing A-Check Maintenance Scheduling with Increased Complexity
Consider an airline that needs to optimize its A-check maintenance schedule to balance aircraft availability and operational safety. The airline wants to know:
- What if they reduce the frequency of A-checks by extending the interval by 10%?
- What if additional technicians are allocated to speed up inspections?
- What if unexpected delays in spare part delivery occur?
- What if new regulations require additional safety checks?
- What if fuel price changes affect overall operational costs?
- What if certain technicians are not available due to sick leave or unexpected events?
- What if new equipment requires specialized training, affecting technician productivity?
Using Decision Trees, we can model the impact of these changes on aircraft availability, operational costs, technician workloads, spare part logistics, and the risk of unplanned maintenance.
Here's an implementation using Python and scikit-learn, presented in a more understandable way for non-technical users:
# Import necessary libraries
import pandas as pd
from sklearn.tree import DecisionTreeRegressor, plot_tree
import matplotlib.pyplot as plt
# Creating a simple dataset to model A-check maintenance scenarios
# Data includes A-check intervals, number of technicians, delays in spare part delivery, and more
maintenance_data = {
'A_Check_Interval_Days': [60, 70, 80, 90, 100], # How often maintenance is performed (in days)
'Technicians_Assigned': [5, 6, 7, 8, 9], # Number of technicians available
'Part_Delivery_Delay_Hours': [0, 5, 10, 15, 20], # Delay in getting spare parts (in hours)
'Additional_Safety_Checks': [0, 1, 0, 1, 0], # Whether additional safety checks are required (0 or 1)
'Fuel_Price_Impact': [1.1, 1.2, 1.3, 1.5, 1.7], # Impact of fuel price changes
'Technician_Availability': [1, 0.9, 0.8, 0.95, 0.85], # Availability of technicians (1 means full availability)
'Aircraft_Downtime_Hours': [100, 110, 115, 120, 125] # Resulting aircraft downtime in hours
}
# Convert the data to a table format
maintenance_df = pd.DataFrame(maintenance_data)
# Define features that impact downtime (inputs) and the target (output)
features = maintenance_df[['A_Check_Interval_Days', 'Technicians_Assigned', 'Part_Delivery_Delay_Hours',
'Additional_Safety_Checks', 'Fuel_Price_Impact', 'Technician_Availability']]
target = maintenance_df['Aircraft_Downtime_Hours']
# Build a Decision Tree model to predict aircraft downtime
model = DecisionTreeRegressor(max_depth=5, random_state=42)
model.fit(features, target)
# Visualize the decision-making process in an easy-to-understand diagram
plt.figure(figsize=(16, 12))
plot_tree(model, feature_names=features.columns, filled=True)
plt.show()
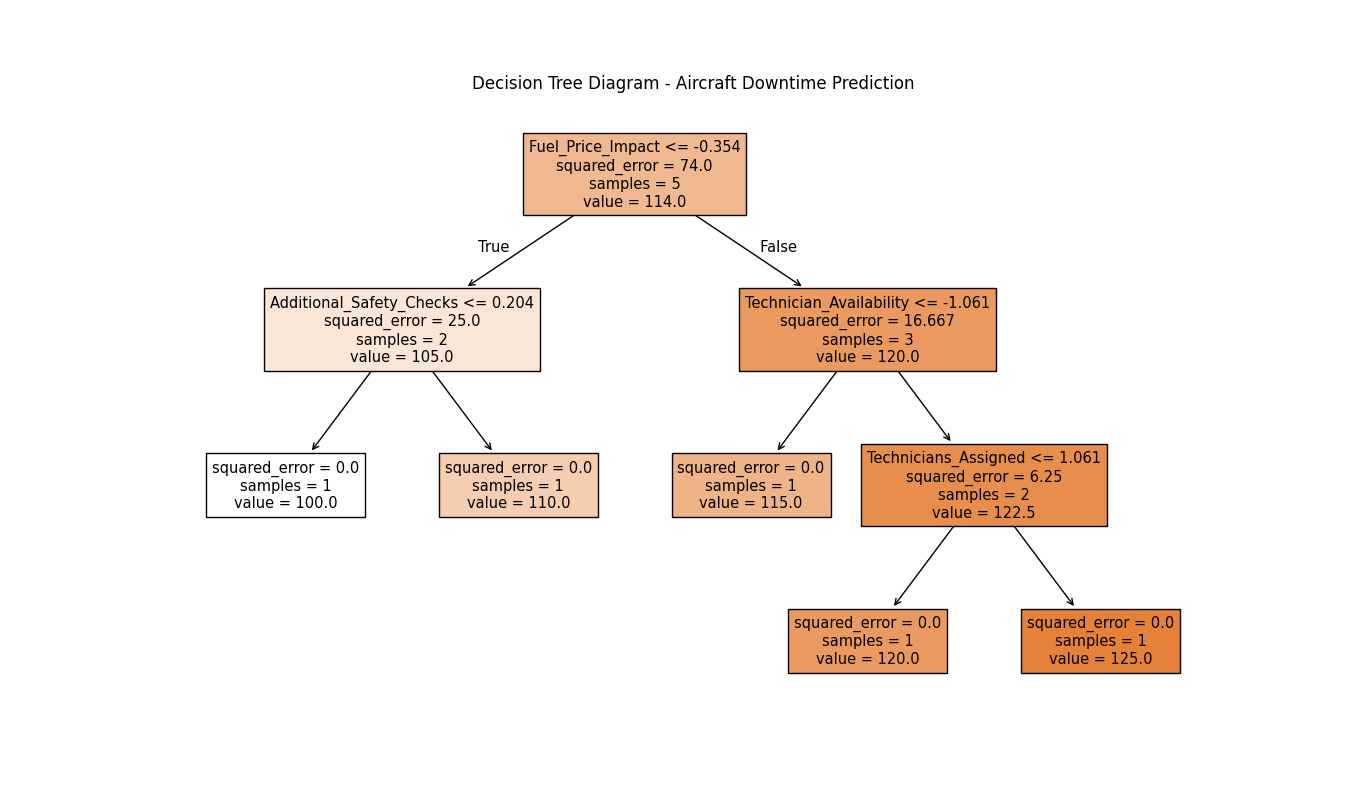
In the above example, we create a simple table that includes the following factors:
- A_Check_Interval_Days: How frequently the A-check maintenance is performed (e.g., every 60, 70, or 80 days).
- Technicians_Assigned: Number of technicians assigned to perform the maintenance.
- Part_Delivery_Delay_Hours: How long it takes for spare parts to arrive (in hours).
- Additional_Safety_Checks: Whether any extra safety checks are required (either 0 for no or 1 for yes).
- Fuel_Price_Impact: How changes in fuel prices impact overall operational costs.
- Technician_Availability: The percentage of technician availability (e.g., 1 means all technicians are available).
The Decision Tree Regressor helps predict the expected aircraft downtime based on different combinations of these variables. The decision tree diagram visually shows the flow of decisions made by the model to predict downtime, making it more accessible for non-technical stakeholders to understand.
Using the Decision Tree for MRO What-if Analysis
Once we have our Decision Tree model, we can simulate different maintenance scenarios with greater granularity:
What if we extend the A-check interval by 10% and there are part delivery delays?
new_scenario = pd.DataFrame({
'A_Check_Interval_Days': [90 * 1.1], # Extend by 10%
'Technicians_Assigned': [8],
'Part_Delivery_Delay_Hours': [10],
'Additional_Safety_Checks': [1],
'Fuel_Price_Impact': [1.5],
'Technician_Availability': [0.95]
})
predicted_downtime = model.predict(new_scenario)
print(f"Predicted Aircraft Downtime with extended A-check interval and part delivery delays: {predicted_downtime[0]} hours")
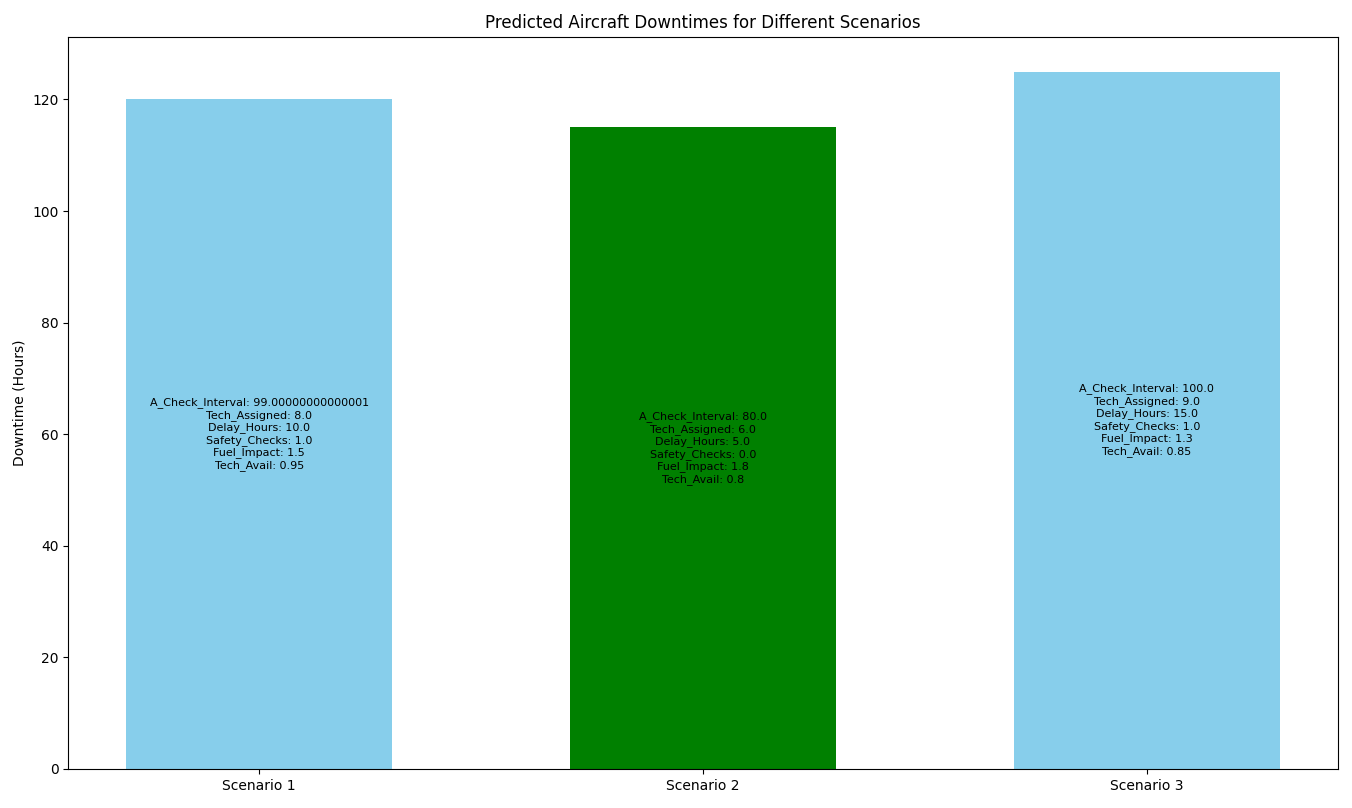
What if fuel prices increase and fewer technicians are available?
new_scenario = pd.DataFrame({
'A_Check_Interval_Days': [80],
'Technicians_Assigned': [6],
'Part_Delivery_Delay_Hours': [5],
'Additional_Safety_Checks': [0],
'Fuel_Price_Impact': [1.8], # Increased fuel price
'Technician_Availability': [0.8] # Fewer technicians available
})
predicted_downtime = model.predict(new_scenario)
print(f"Predicted Aircraft Downtime with increased fuel prices and reduced technician availability: {predicted_downtime[0]} hours")

These scenarios help MRO planners determine the optimal balance between maintenance frequency, technician allocation, spare part logistics, regulatory requirements, and other operational complexities, ensuring minimal disruption to flight schedules.
Feature Importance in MRO Decision-Making
Understanding which factors have the greatest impact on aircraft downtime is crucial for MRO decision-making. Feature importance in a Decision Tree helps identify these key variables, enabling MRO teams to focus their efforts where they matter most.
# Feature importance analysis
importances = model.feature_importances_
feature_importance_df = pd.DataFrame({
'Feature': features.columns,
'Importance': importances
}).sort_values(by='Importance', ascending=False)
print(feature_importance_df)
This analysis provides insights into whether increasing the number of technicians, adjusting the maintenance interval, addressing part delivery delays, focusing on safety checks, or managing fuel price impacts has the greatest influence on minimizing downtime, thus informing strategic decisions.
Advanced Scenario Exploration for MRO Operations
For MRO staff who may not be familiar with reading complex decision charts, advanced machine learning can be integrated with AI chat technology to bridge this gap. Instead of interpreting visual decision trees, users can simply interact with different variables, and receive straightforward answers like "Predicted Aircraft Downtime with increased fuel prices and reduced technician availability: 115.0 hours". This makes powerful analysis accessible to all, regardless of technical expertise.
What-if Analysis becomes even more impactful when integrated with visualization tools like Plotly or Power BI. By creating interactive dashboards, MRO planners can adjust variables like maintenance intervals, technician assignments, spare part availability, and safety checks in real time, immediately visualizing the impact on aircraft availability and operational costs.
At ePlaneAI, we build custom ML models, data-intensive dashboards that allow granular exploration of scenarios or integrate with existing cloud-based dashboard solutions to bring all your data into one place. Our goal is to provide MRO teams with powerful tools for decision-making that drive efficiency and minimize costs.
For example, using Dash by Plotly or Qlik, you could create a web application that allows MRO managers to explore different maintenance schedules, technician allocations, fuel price impacts, and other variables while visualizing the predicted aircraft downtime.
Deploying MRO What-if Analysis in the Cloud
Deploying What-if Analysis solutions in the cloud brings scalability to MRO operations. Using cloud services like AWS Lambda or Azure Functions, Decision Tree models can be deployed as serverless functions that accept input scenarios and return maintenance predictions in real time.
Here’s an example of setting up an AWS Lambda function for What-if Analysis in MRO:
import json
import joblib
import pandas as pd
from sklearn.tree import DecisionTreeRegressor
# Load the model (assuming it's been trained and saved)
def load_model():
try:
model = joblib.load('/tmp/decision_tree_model.pkl')
except FileNotFoundError:
raise Exception("Model file not found. Ensure the path is correct.")
return model
def lambda_handler(event, context):
# Parse input scenario data
try:
input_data = json.loads(event['body'])
except (KeyError, json.JSONDecodeError) as e:
return {
'statusCode': 400,
'body': json.dumps({'error': 'Invalid input format', 'details': str(e)})
}
# Load pre-trained Decision Tree model
try:
model = load_model()
except Exception as e:
return {
'statusCode': 500,
'body': json.dumps({'error': 'Failed to load model', 'details': str(e)})
}
# Convert input to DataFrame
new_data = pd.DataFrame([input_data])
# Predict outcome using the Decision Tree model
try:
prediction = model.predict(new_data)
return {
'statusCode': 200,
'body': json.dumps({'predicted_downtime': prediction[0]})
}
except Exception as e:
return {
'statusCode': 500,
'body': json.dumps({'error': 'Prediction failed', 'details': str(e)})
}
Conclusion
What-if Analysis combined with Decision Trees provides MRO teams in the aviation industry with the tools needed to explore different maintenance scenarios, optimize technician schedules, and make data-driven decisions. By understanding the impact of different choices, airlines and MRO providers can ensure that aircraft are maintained efficiently, minimizing downtime and keeping operations running smoothly.
At ePlaneAI, we specialize in providing tailored solutions to help you navigate the complexities of MRO operations with confidence. Whether you need a custom-built data dashboard or integration with your existing cloud-based solutions, we are here to help. If you're interested in exploring how What-if Analysis can transform your MRO operations, let's connect and take aviation maintenance to new heights.